Oh noes!
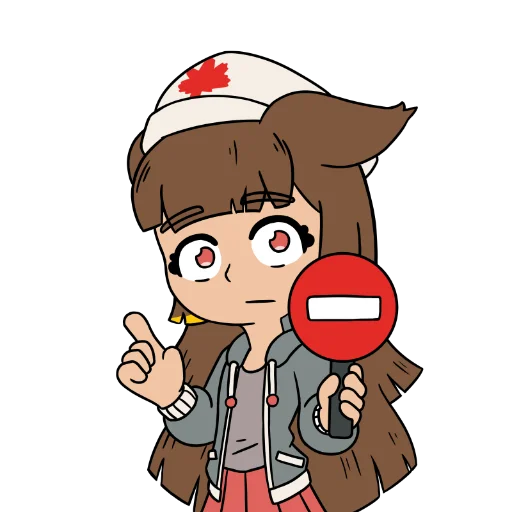
Access Denied: error code 26301432c34cf028.
Go home or if you believe you should not be blocked, please contact the webmaster at git@puqns67.icu
Access Denied: error code 26301432c34cf028.
Go home or if you believe you should not be blocked, please contact the webmaster at git@puqns67.icu